The following code adds a custom price input field on the product page for a specific product (product ID 38) and sets the entered custom price as the product price in the cart and checkout.
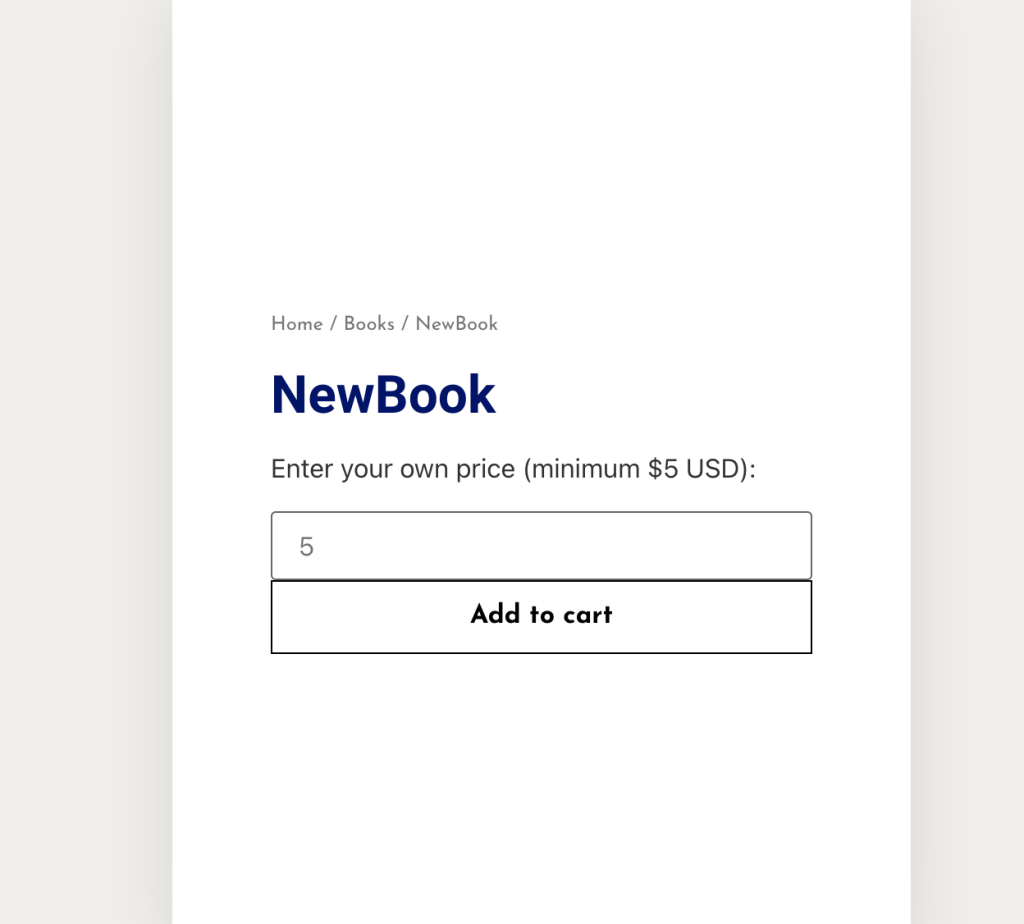
The add_custom_price_field()
the function adds the custom price input field before the add to cart button on the product page if the product ID is 38.
The set_custom_cart_item_data()
the function sets the custom price as cart item data if the custom price input field is set and not empty, and the product ID is 38. It enforces a minimum price of $5 USD.
The set_custom_cart_item_price()
the function sets the custom price as the product price in the cart and checkout for cart items that have the custom price cart item data.
Overall, this code allows customers to set their own price for a specific product and ensures that the custom price is used as the product price throughout the checkout process.
Add a Custom Price Field above Add to cart button
add_action( 'woocommerce_before_add_to_cart_button', 'add_custom_price_field', 10 );
function add_custom_price_field() {
global $product;
if ( $product->get_id() == 38 ) {
echo '<div class="custom-price">';
echo '<p>' . __('Enter your own price (minimum $5 USD):') . '</p>';
echo '<input type="number" step="1" min="5" name="custom_price" id="custom_price" placeholder="5" value="" required />';
echo '</div>';
}
}
add_filter( 'woocommerce_add_cart_item_data', 'set_custom_cart_item_data', 10, 2 );
function set_custom_cart_item_data( $cart_item_data, $product_id ) {
if ( isset( $_POST['custom_price'] ) && ! empty( $_POST['custom_price'] ) && $product_id == 38 ) {
$custom_price = floatval( wc_clean( $_POST['custom_price'] ) );
$custom_price = max( 5, $custom_price ); // enforce minimum price of 5 USD
$cart_item_data['custom_price'] = $custom_price;
}
return $cart_item_data;
}
// Set custom price as product price in cart and checkout
add_action( 'woocommerce_before_calculate_totals', 'set_custom_cart_item_price', 10 );
function set_custom_cart_item_price( $cart ) {
if ( is_admin() && ! defined( 'DOING_AJAX' ) ) {
return;
}
foreach ( $cart->get_cart() as $cart_item_key => $cart_item ) {
if ( isset( $cart_item['custom_price'] ) ) {
$cart_item['data']->set_price( $cart_item['custom_price'] );
}
}
}